Diameter of Binary Tree
franklinqin0 TreeDFS
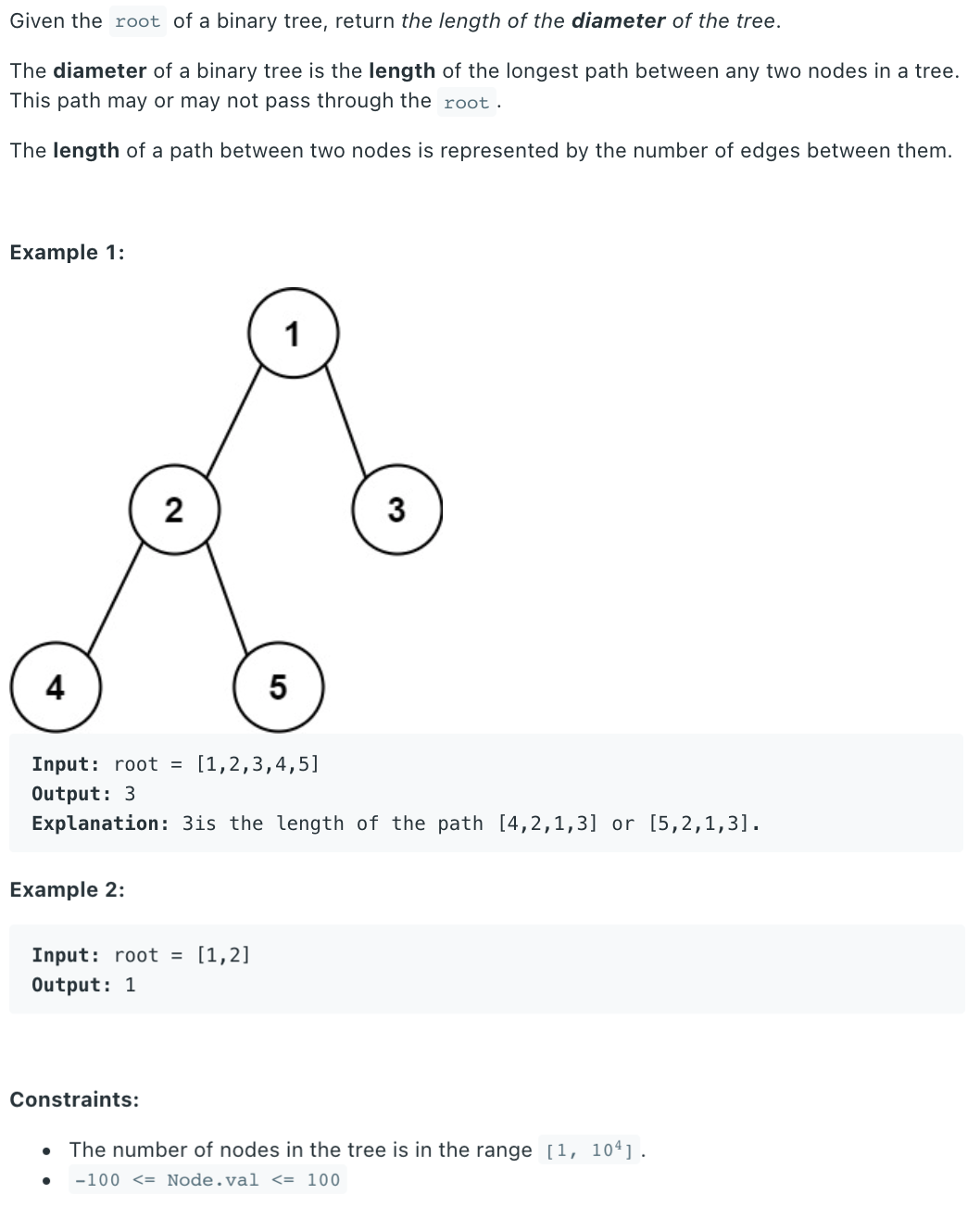
# Definition for a Binary Tree Node
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
1
2
3
4
5
2
3
4
5
# Solution
Let be the number of nodes in the tree.
# DFS
Complexity
time:
space:
def diameterOfBinaryTree(self, root: TreeNode) -> int:
res = 0
def longest_path(node):
if not node:
return 0
nonlocal res
# recursively find the longest path in left and right child
left_path = longest_path(node.left)
right_path = longest_path(node.right)
# update the diameter if left_path + right_path is larger
res = max(res, left_path + right_path)
# return the longer one btw left_path and right_path
# add 1 for the path connecting the node and its parent
return max(left_path, right_path) + 1
longest_path(root)
return res
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20