Jump Game
franklinqin0 ArrayGreedy
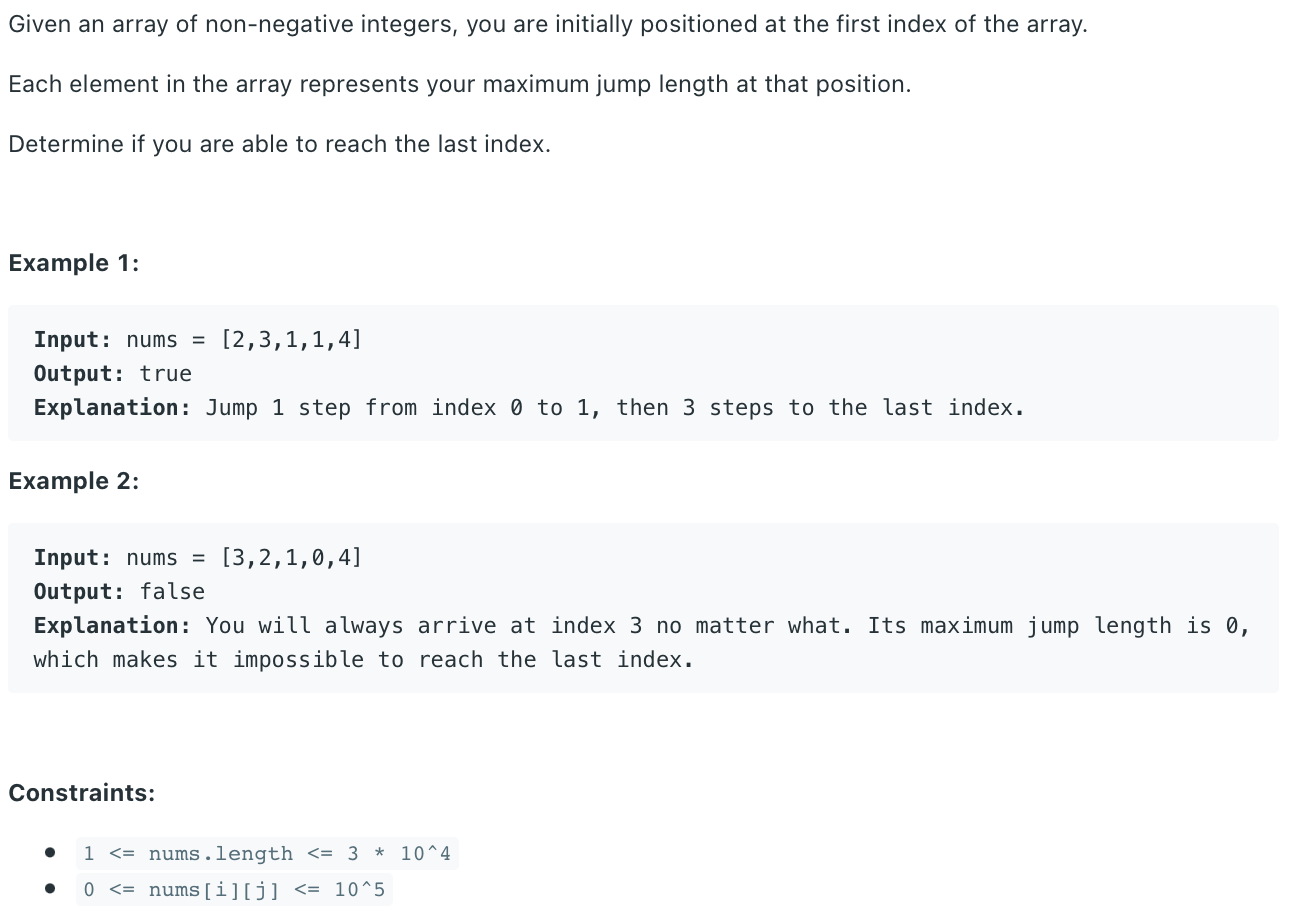
# Solution
Let be the length of the array nums
.
# Greedy Algorithm
Complexity
time:
space:
# Front to Back
def canJump(self, nums: List[int]) -> bool:
n = len(nums)
max_right = 0
for i in range(n):
# if i can be reached, update max_right
if max_right >= i:
max_right = max(max_right, i + nums[i])
# if max_right can reach last element, can jump
if max_right >= n-1:
return True
return False
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
# Back to Front
def canJump(self, nums: List[int]) -> bool:
n = len(nums)
max_left = n-1
for i in reversed(range(n)):
# if position i can advance to max_left, update max_left to i
if max_left <= i + nums[i]:
max_left = i
# if max_left is not at 0, cannot jump
return max_left == 0
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9