Find All Numbers Disappeared in an Array
franklinqin0 ArrayHash Table
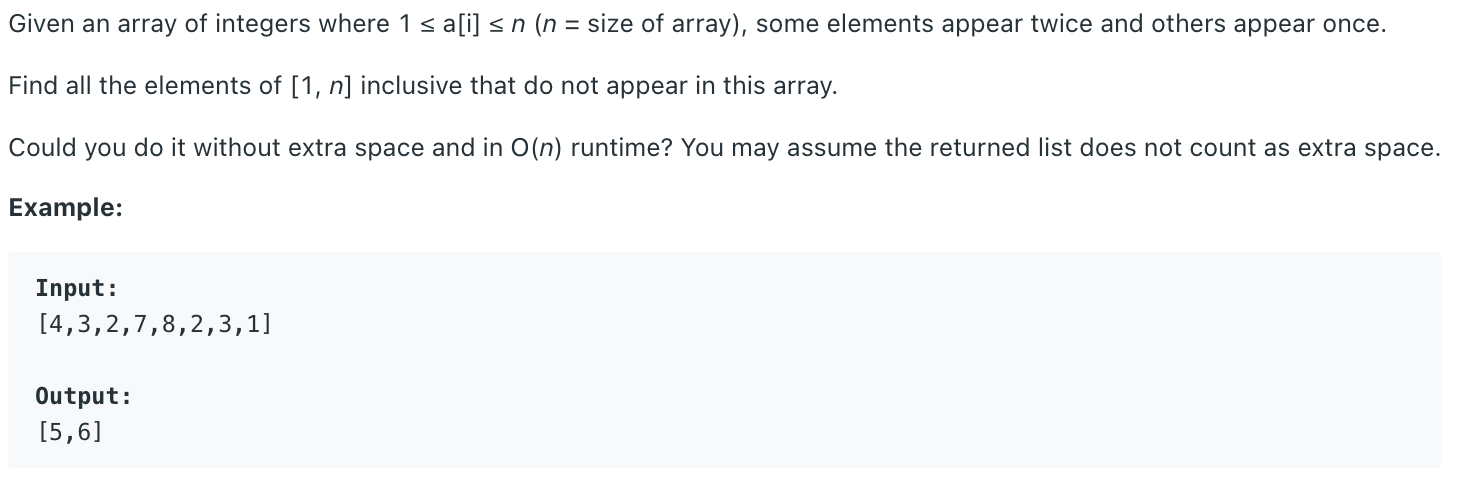
# Solution
Let be the length of the array.
# 1-liner Cheating
from collections import Counter
def findDisappearedNumbers(self, nums: List[int]) -> List[int]:
return Counter(list(range(1,len(nums)+1))) - Counter(nums)
1
2
3
2
3
# HashMap
The trick is to turn nums
into a HashMap, w/ mapping btw (num-1)%n
and whether num
has occurred.
Complexity
time:
space:
def findDisappearedNumbers(self, nums: List[int]) -> List[int]:
n = len(nums)
res = []
for num in nums:
# establish a mapping
nums[(num-1)%n] += n
for i in range(n):
# number i+1 was not encountered and not in nums
if nums[i] <= n:
res.append(i+1)
return res
1
2
3
4
5
6
7
8
9
10
11
12
13
14
2
3
4
5
6
7
8
9
10
11
12
13
14