Intersection of Two Linked Lists
franklinqin0 Linked ListTwo Pointers
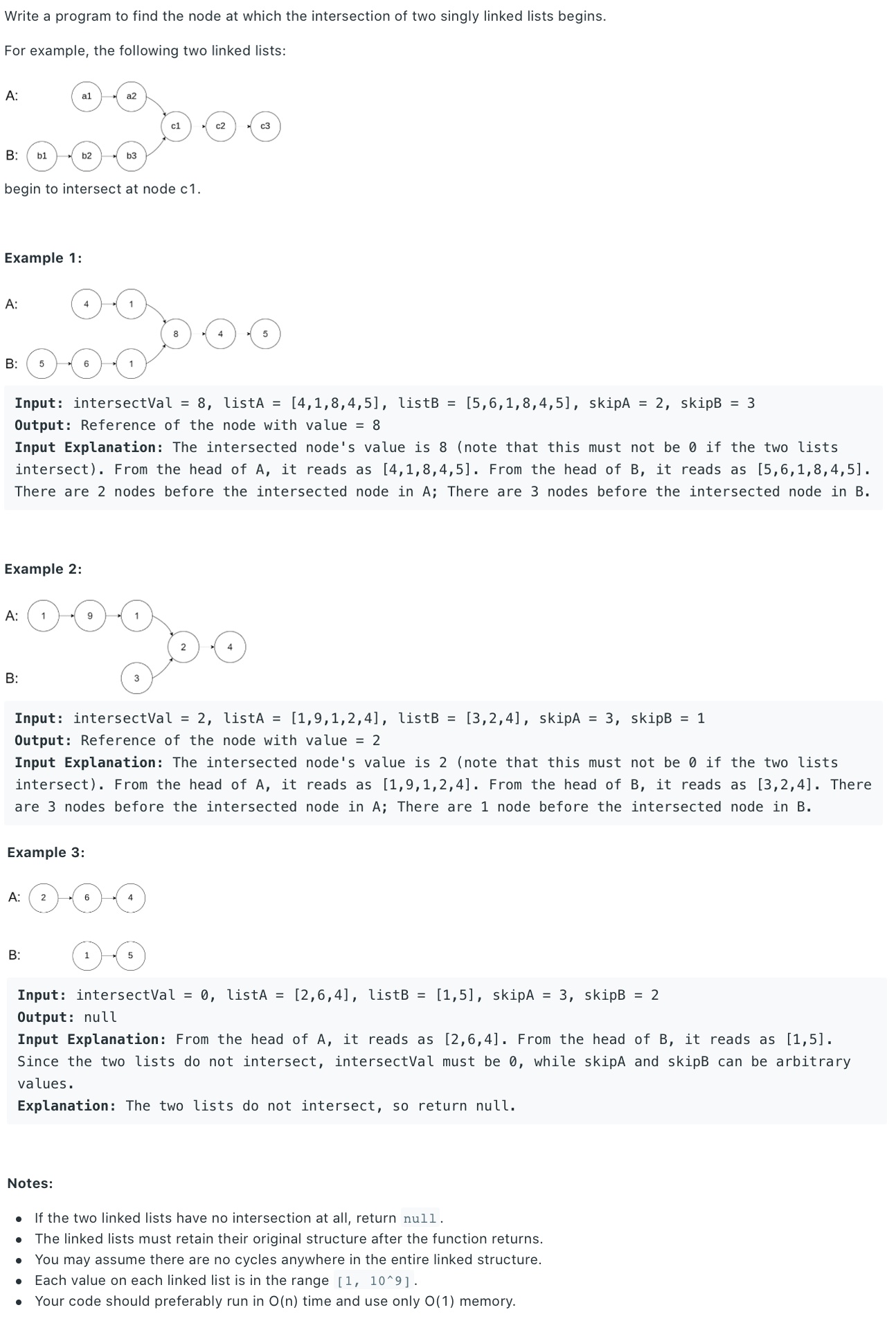
# Definition for Singly-Linked List
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
1
2
3
4
2
3
4
# Solution
Let be the length of list , and be the length of list .
The brute force solution of squared time complexity is omitted.
# HashSet
Complexity
time:
space:
def getIntersectionNode(self, headA: ListNode, headB: ListNode) -> ListNode:
setA = set()
pA = headA
while pA:
setA.add(pA)
pA = pA.next
# return pB is in setA
pB = headB
while pB:
if pB in setA:
return pB
pB = pB.next
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12
# Two Pointers
Complexity
time:
space:
If you switch head, the possible difference between length would be counted. On the second traversal, pA
and pB
either hit or miss. If they meet, either node can be returned. Otherwise, they will hit at the end in the 2nd iteration, pA == pB == None
, and return either one of them is the same: None
.
def getIntersectionNode(self, headA: ListNode, headB: ListNode) -> ListNode:
pA = headA
pB = headB
while pA is not pB:
pA = pA.next if pA is not None else headB
pB = pB.next if pB is not None else headA
return pA
1
2
3
4
5
6
7
2
3
4
5
6
7