Path Sum III
franklinqin0 TreeDFS
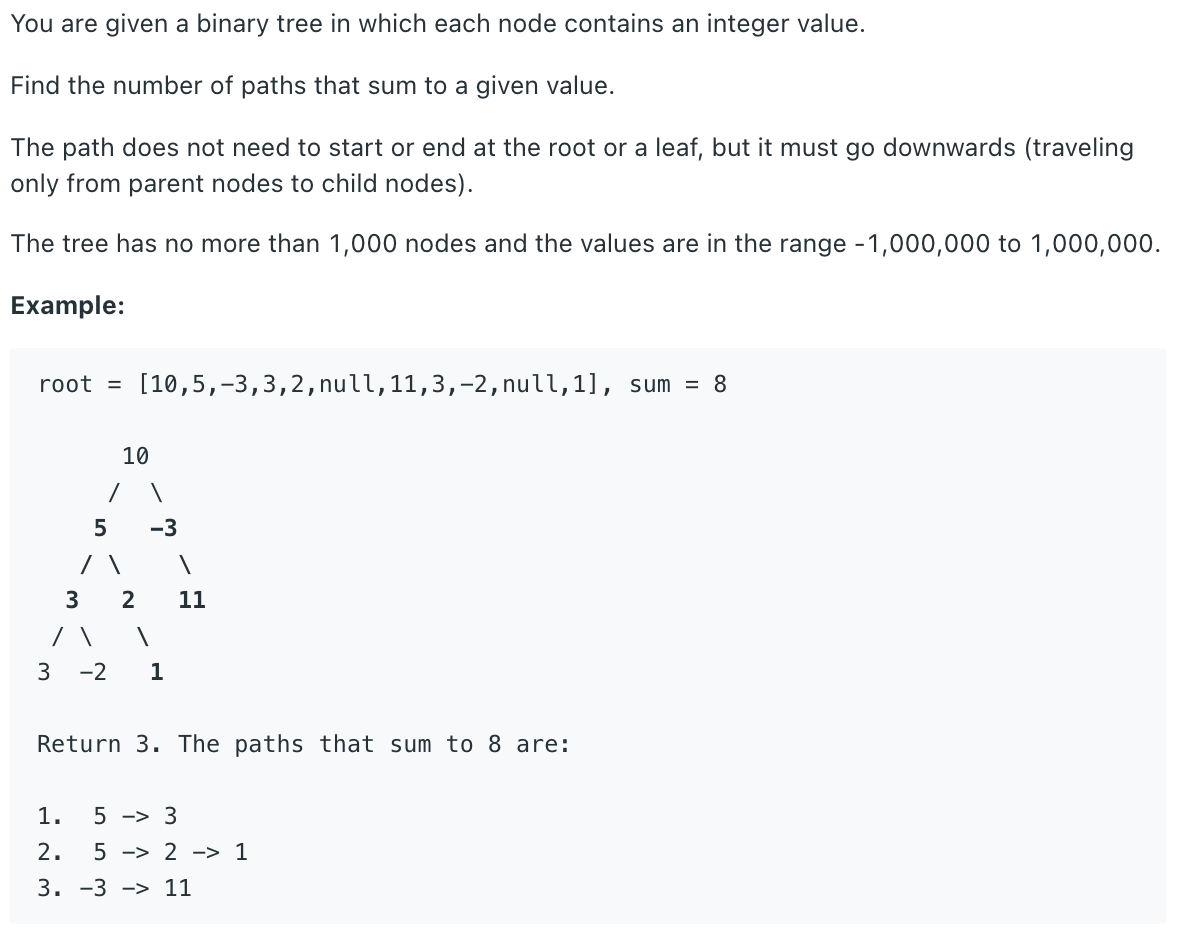
# Definition for a Binary Tree Node
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
1
2
3
4
5
2
3
4
5
# Solution
# Vanilla DFS
Complexity
time: (worst case if tree is a line: )
space: (due to implicit stack space, worst case: )
def pathSum(self, root: TreeNode, sum: int) -> int:
if not root:
return 0
def dfs(node: TreeNode, csum: int) -> int:
if not node:
return 0
csum += node.val
return (csum == sum) + dfs(node.left, csum) + dfs(node.right, csum)
return dfs(root, 0) + self.pathSum(root.left, sum) + self.pathSum(root.right, sum)
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
# Recursive DFS w/ Memoization
Say the current node
has a path sum csum
, if there exists a node that had been visited and had a path sum csum - sum
, then increment res
by memo[csum - sum]
.
As a node
's subtree is being traversed, increment memo[csum]
by .
Complexity
time:
space:
from collections import defaultdict
def pathSum(self, root: TreeNode, sum: int) -> int:
res = 0
memo = defaultdict(int)
memo[0] = 1
def dfs(node, csum):
nonlocal res
if not node:
return
csum += node.val
# increment by the # of times
res += memo[csum - sum]
# mark the current node as visited
memo[csum] += 1
dfs(node.left, csum)
dfs(node.right, csum)
memo[csum] -= 1
dfs(root, 0)
return res
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21