Longest Substring Without Repeating Characters
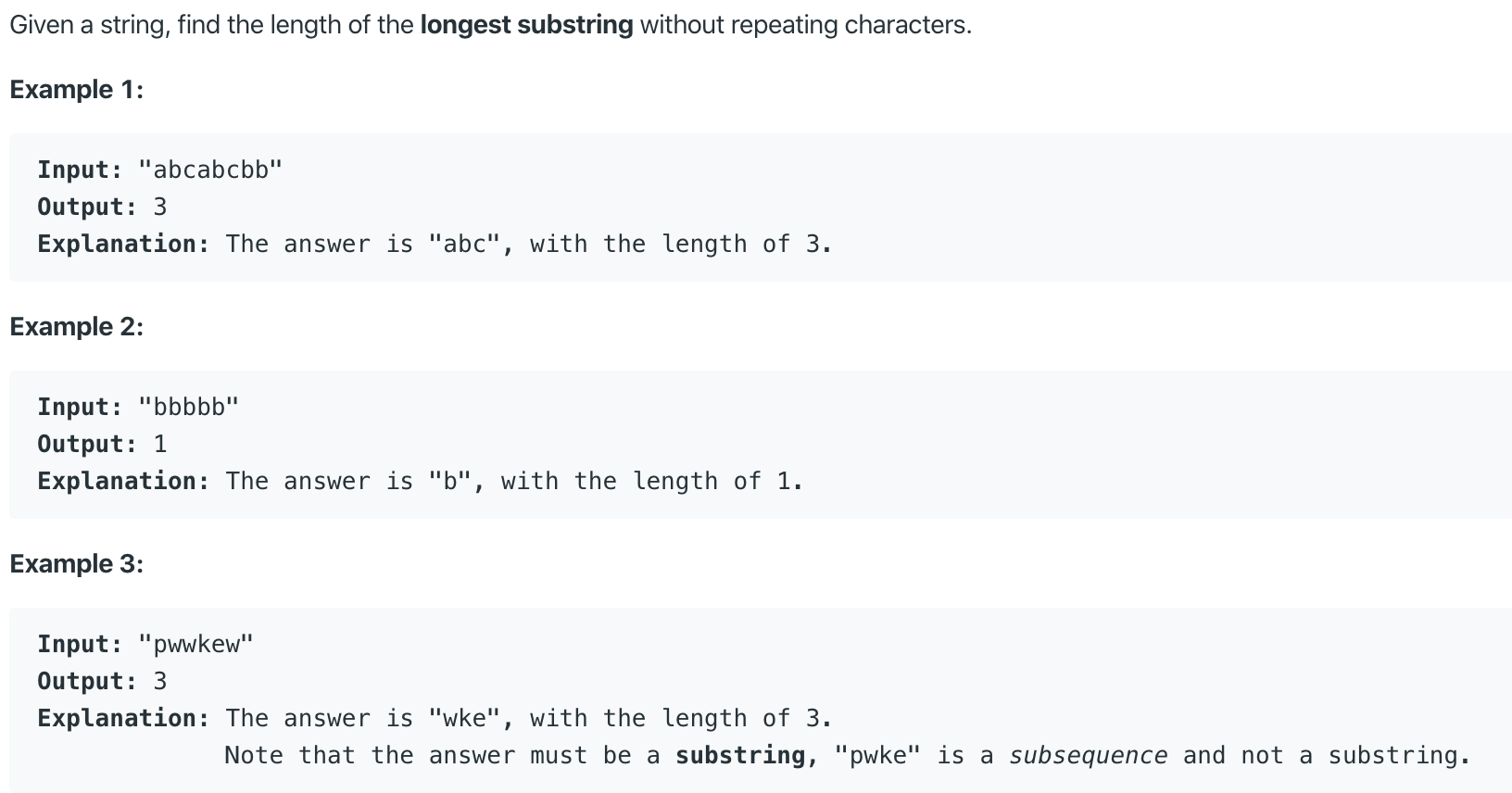
# Solution
Let be size of the string, the size of the charset/alphabet, and the size of the hashset, which is upper bounded by and .
# Brute Force
The brute force solution is not shown.
Complexity
time: (a nested for loop for the sliding window and to check if unique takes time)
space: ( space for the sliding window)
# Sliding Window Using HashSet
The idea is to use a sliding window to locate a substring, and a hashset
to see if the new char is already seen previously.
Sliding window logic: i
is the left boundary and j
the right boundary. Increase j
by 1 if s[j]
has not occurred in the current subarray. Increase i
if s[j]
has occurred.
Invariant: i
<=j
Complexity
time: (worst case: , all characters are the same and each will be visited by both and )
space:
def lengthOfLongestSubstring(self, s: str) -> int:
if not s: return 0
n = len(s)
hashset = set()
i = j = 0
res = 1
while i < n and j < n:
if s[j] not in hashset:
hashset.add(s[j])
j += 1
res = max(res, j-i)
else:
hashset.remove(s[i])
i += 1
return res
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# Sliding Window Using HashMap
If having seen the new char in existing window, could update the left boundary to d[val]+1
( + index of last duplicate in the window).
Several optimizations:
start
andidx
rather thani
andj
to refer to left and right boundaries of windowenumerate
idx
andval
dct
to store the mapping fromval
toidx
Complexity
time:
space:
def lengthOfLongestSubstring(self, s: str) -> int:
res = 0
start = 0
dct = {}
for idx, val in enumerate(s):
if s[idx] in dct:
start = max(start, dct[val]+1)
res = max(res, idx-start+1)
dct[val] = idx
return res
2
3
4
5
6
7
8
9
10