Plus One Linked List
franklinqin0 Linked List
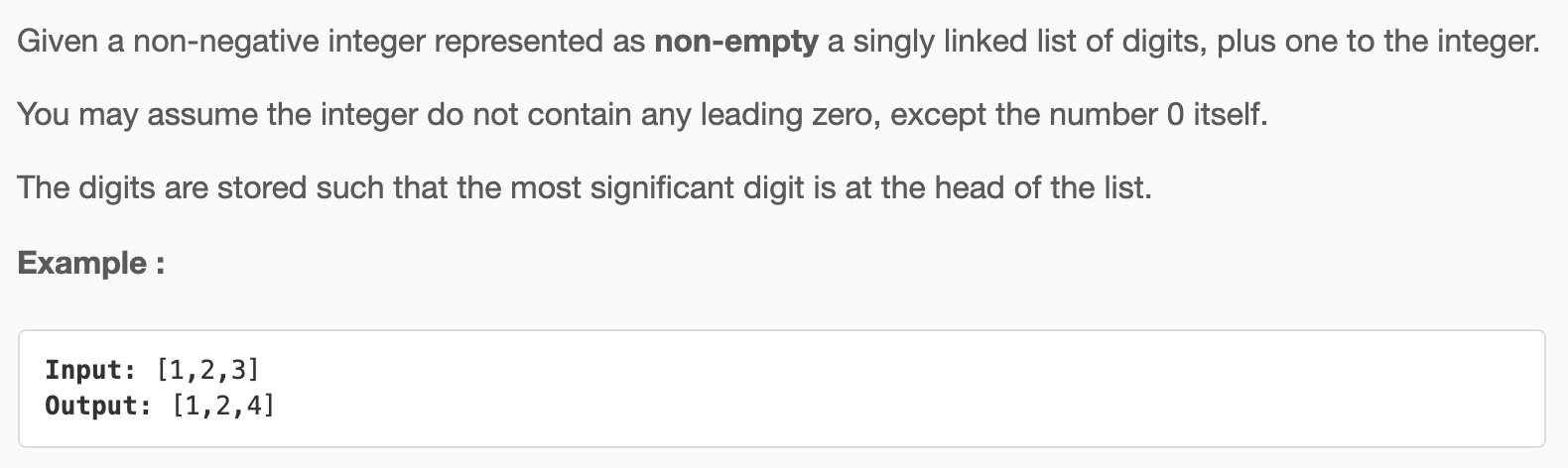
# Solution
There are many other possible solutions, but none is as good as the following one.
Let be the length of linked list.
# Rightmost Not-Nine Digit
Note:
sentinel
node is set to1
for number is all9
's and otherwise0
not-nine
digit means all the following digits are . So the not-nine digit in2->9->1->null
is1
, and in0->9->9
is sentinel0
Complexity
time:
space:
def plusOne(self, head):
sentinel = ListNode(0)
sentinel.next = head
# find the rightmost digit != 9
not_nine = sentinel
while head:
if head.val != 9:
not_nine = head
head = head.next
# increase this rightmost not-nine digit by 1
not_nine.val += 1
not_nine = not_nine.next
# set all the following nines to zeros
while not_nine:
not_nine.val = 0
not_nine = not_nine.next
return sentinel if sentinel.val else sentinel.next
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20