Robot Bounded In Circle
franklinqin0 MathString
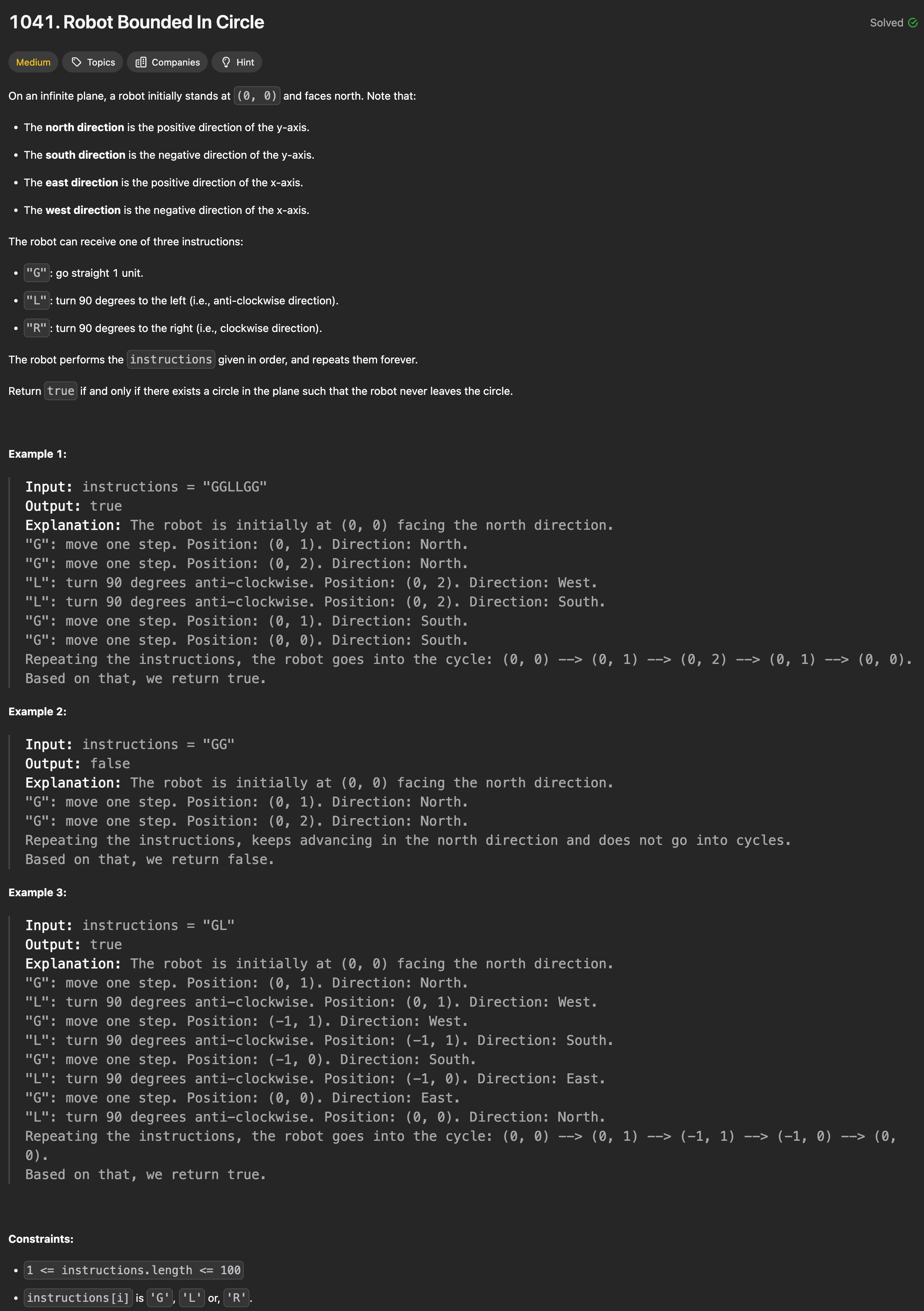
# Solution
class Solution(object):
def __init__(self):
self.pos = [0, 0]
self.dir_idx = 0
self.directions = ["N", "W", "S", "E"]
self.dir = "N"
def update_dir(self, ins):
if ins == "L":
self.dir_idx += 1
elif ins == "R":
self.dir_idx -= 1
self.dir_idx %= 4
self.dir = self.directions[self.dir_idx]
def update_pos(self):
if self.dir == "N":
self.pos[1] += 1
elif self.dir == "S":
self.pos[1] -= 1
elif self.dir == "W":
self.pos[0] -= 1
elif self.dir == "E":
self.pos[0] += 1
def execute_instructions(self, instructions):
for ins in instructions:
if ins == "G":
self.update_pos()
elif ins == "L" or ins == "R":
self.update_dir(ins)
def isRobotBounded(self, instructions):
"""
:type instructions: str
:rtype: bool
"""
# either robot changes direction (not stay pointing north), or it doesn't move
self.execute_instructions(instructions)
if self.pos == [0, 0] or self.dir != "N":
return True
return False
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44