Hamming Distance
franklinqin0 Bit
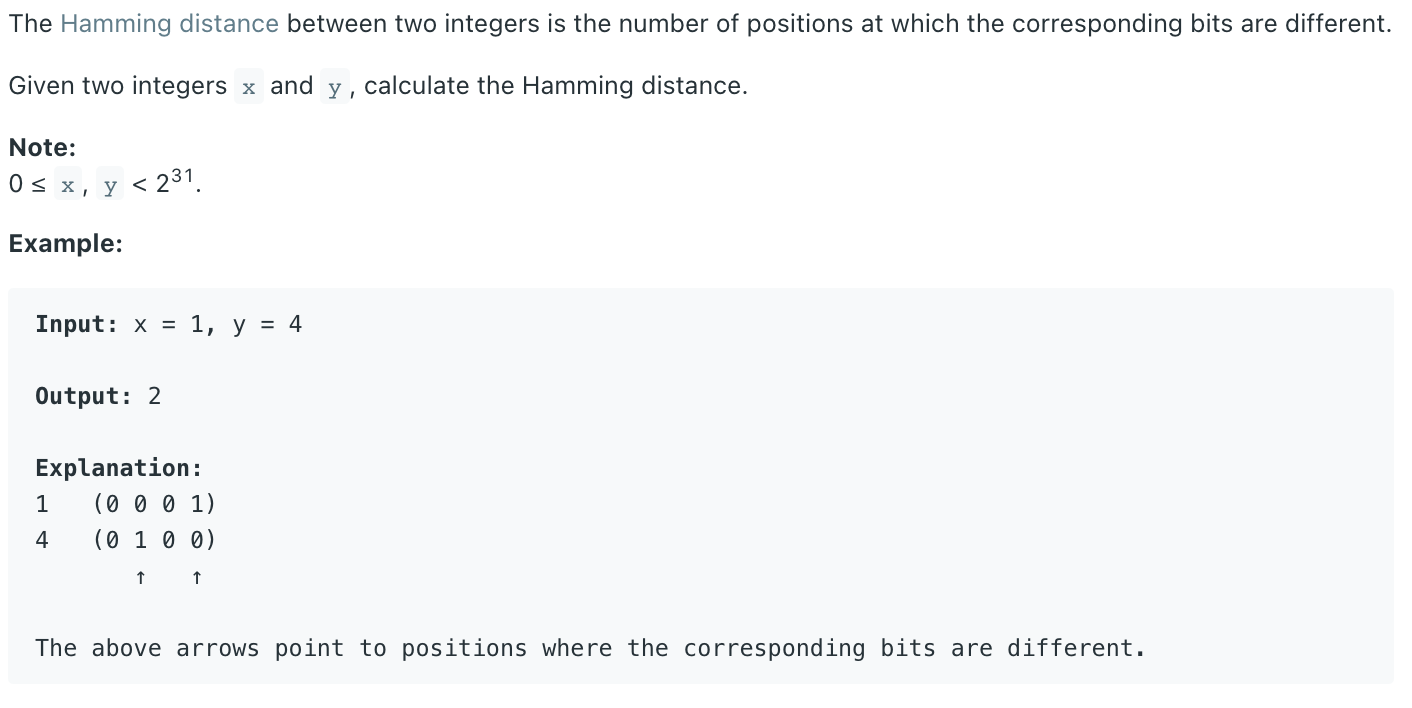
# Solution
As quoted from the problem statement:
The Hamming distance (opens new window) between two integers is the number of positions at which the corresponding bits are different.
The problem solution consists of 2 parts:
- calculate the
xor
ofx
andy
- count the number of bits in the
xor
The space to store int
is constant.
Complexity
time:
space:
# 1-liner Cheating
def hammingDistance(self, x: int, y: int) -> int:
return bin(x ^ y).count('1')
1
2
2
# Least Significant Bit
def hammingDistance(self, x: int, y: int) -> int:
xor = x ^ y
res = 0
while xor:
if xor & 1 > 0:
res += 1
xor >>= 1
return res
1
2
3
4
5
6
7
8
9
10
2
3
4
5
6
7
8
9
10
# Brian Kernighan algorithm
Counting the lowest set bit is faster than counting the least significant set bits.
def hammingDistance(self, x: int, y: int) -> int:
xor = x ^ y
res = 0
while xor:
res += 1
xor = xor & (xor-1)
return res
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9