Reverse Linked List
franklinqin0 Linked ListRecursion
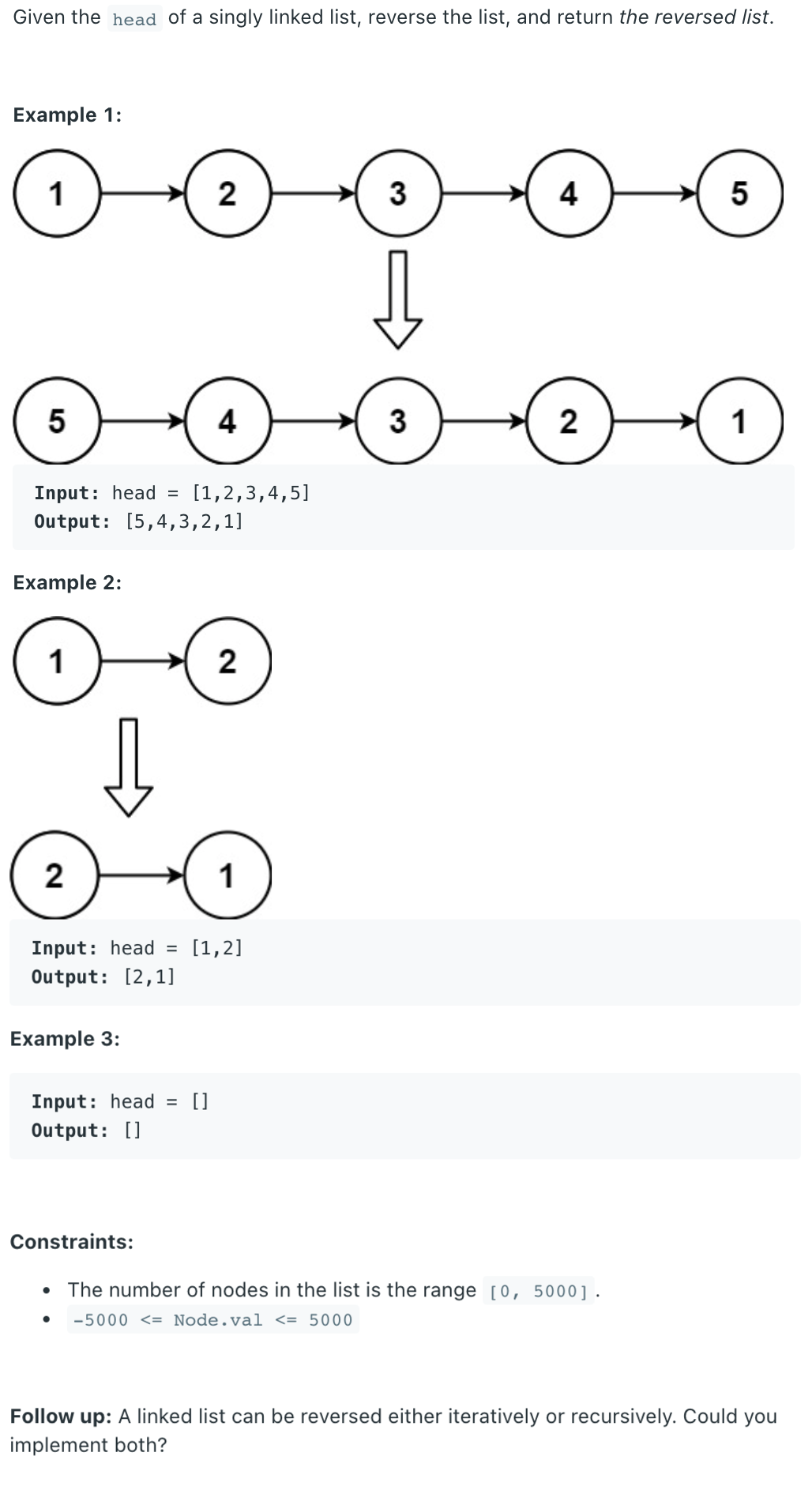
# Definition for Singly-Linked List
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
1
2
3
4
2
3
4
# Solution
This problem is very basic yet IMPT. It can be solved both iteratively or recursively.
# Iteration
It's quite IMPT to understand and remember the steps in while loop.
Complexity
time:
space:
def reverseList(self, head: ListNode) -> ListNode:
prev = None
curr = head
while curr:
# store next node
nxt = curr.next
# update next of current
curr.next = prev
# move prev and curr 1 step forward
prev = curr
curr = nxt
return prev
1
2
3
4
5
6
7
8
9
10
11
12
2
3
4
5
6
7
8
9
10
11
12
or could combine the assignments (Python's syntactic sugar):
def reverseList(self, head: ListNode) -> ListNode:
prev, curr = None, head
while curr:
curr.next, prev, curr = prev, curr, curr.next
return prev
1
2
3
4
5
2
3
4
5
# Follow Up
A linked list can be reversed either iteratively or recursively. Could you implement both?
# Recursion (REDO)
There are n
nodes in total. Assume to have been reversed and the curr
node is :
Set 's next
to : .next.next
=
Also avoid the cycle by setting 's next
to None
: .next
= None
Note that I set head
to curr
to avoid confusion. hd
is the actual head of reversed linked list.
Complexity
time:
space: (due to implicit stack space)
def reverseList(self, head: ListNode) -> ListNode:
curr = head
# not curr covers the empty input: []
if not curr or not curr.next:
return curr
hd = self.reverseList(curr.next)
curr.next.next = curr
curr.next = None
return hd
1
2
3
4
5
6
7
8
9
2
3
4
5
6
7
8
9