Matrix Restoration
franklinqin0 ArrayPrefix Sum
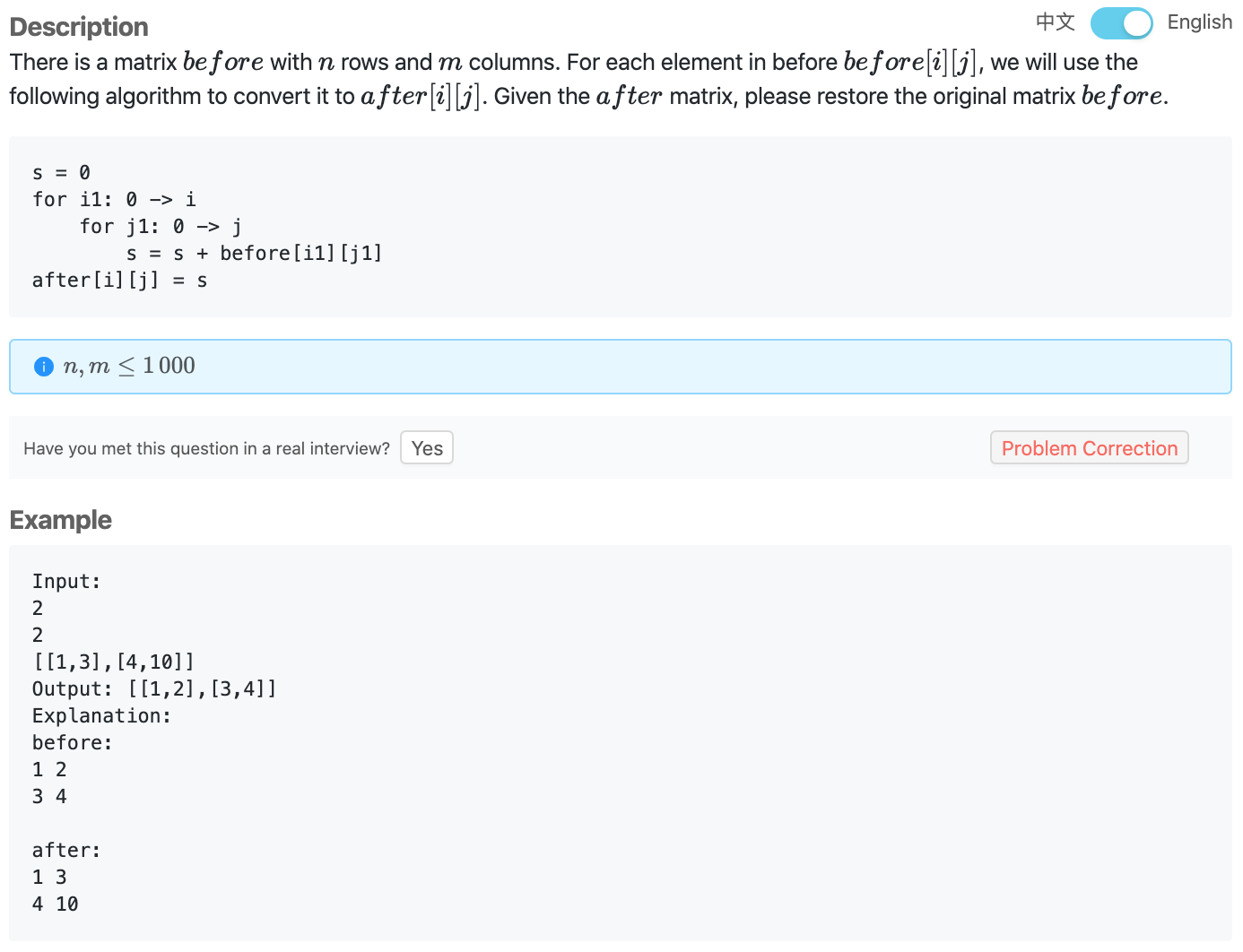
# Solution
Let grid
be before
, and prefix_sum
be after
.
# Prefix Sum
From prefix_sum[i][j] = prefix_sum[i][j-1] + prefix_sum[i-1][j] + grid[i][j] - prefix_sum[i-1][j-1]
, after moving terms we get:
grid[i][j] = prefix_sum[i][j] - prefix_sum[i][j-1] - prefix_sum[i-1][j] + prefix_sum[i-1][j-1]
def matrixRestoration(self, n, m, prefix_sum):
"""
@param n: the row of the matrix
@param m: the column of the matrix
@param after: the matrix
@return: restore the matrix
"""
grid = [[0 for _ in range(m)] for _ in range(n)]
for i in range(n):
for j in range(m):
grid[i][j] = prefix_sum[i][j]
if i > 0:
grid[i][j] -= prefix_sum[i-1][j]
if j > 0:
grid[i][j] -= prefix_sum[i][j-1]
if i > 0 and j > 0:
grid[i][j] += prefix_sum[i-1][j-1]
return grid
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18